Show Contents Previous Page Next Page
Chapter 7 - Other Request Phases Perl Server-Side Includes
Another feature of mod_perl is that it integrates with the Apache mod_include server-side include (SSI) system. Provided that mod_perl was built with the PERL_SSI option (or with the recommended setting of EVERYTHING=1), the Perl API adds a new #perl element to the standard mod_include server-side include system, allowing server-side includes to call Perl subroutines directly.
The syntax for calling Perl from SSI documents looks like this:
<!--#perl sub="" args=""-->
The tag looks like other server-side include tags but contains the embedded element #perl. The #perl element recognizes two attributes, sub and args. The required sub attribute specifies the subroutine to be invoked. This attribute must occur only once in the tag. It can be the name of any subroutine already loaded into the server (with a PerlModule directive, for instance) or an anonymous subroutine created on the fly. When this subroutine is invoked, it is passed a blessed Apache request object just as if it were a handler for the response phase. Any text that the subroutine prints will appear on the HTML page.
The optional args attribute can occur once or several times in the tag. If present, args attributes specify additional arguments to be passed to the subroutine. They will be presented to the subroutine in the same order in which they occur in the tag.
Example 7-13 shows a simple server-side include
page that uses #perl elements. It has two Perl includes. The simpler
of the two is just a call to a routine named MySSI::remote_host().
When executed, it calls the request object's get_remote_host() method
to fetch the DNS name of the remote host machine:
<!--#perl sub="MySSI::remote_host" -->
MySSI::remote_host() must be preloaded in order for this include to succeed. One way to do this is inside the Perl startup file. Alternatively, it could be defined in a module named MySSI.pm and loaded with the directive PerlModule MySSI. In either case, the definition of remote_host() looks like this:
package MySSI;
sub remote_host {
my $r = shift;
print $r->get_remote_host;
}
You could also define the routine to call the request object's print() method, as in $r->print($r->get_remote_host) . It's your call.
The more complex of the two includes defined in this example calls a Perl subroutine that it creates on the fly. It looks like this:
<!--#perl arg="Hello" arg="SSI" arg="World"
sub="sub {
my($r, @args) = @_;
print qq(@args);
}"
-->
In this case the sub attribute points to an anonymous subroutine defined using the sub {} notation. This subroutine retrieves the request object and a list of arguments, which it simply prints out. Because double quotes are already used to surround the attribute, we use Perl's qq operator to surround the arguments. An equally valid alternative would be to backslash the quotes, as in print \"@args\" .
This tag also has three arg attributes, which are passed, in order of appearance, to the subroutine. The effect is to print the string "Hello SSI World".
In order to try this example out, you'll have to have server-side includes activated. This can be done by uncommenting the following two lines in the standard srm.conf server configuration file:
AddType text/html .shtml
AddHandler server-parsed .shtml
You'll also have to activate the Includes option in the directory
in which the document is located. The final result is shown in Figure 7-4.
Figure 7-4. The page displayed by the example
server-side include document 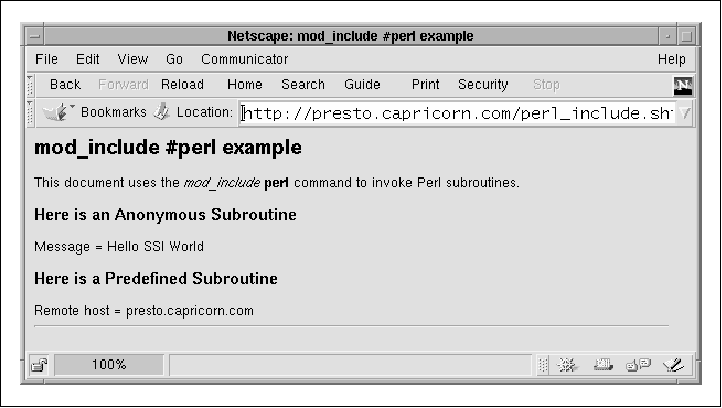 Example 7-13. A Server-Side Include
Document Using #perl Elements
<html>
<!-- file: perl_include.shtml -->
<head>
<title> mod_include #perl example </title>
</head>
<body>
<h1>mod_include #perl example</h1>
This document uses the <i>mod_include</i> <b>perl</b> command to invoke Perl subroutines.
<h3>Here is an Anonymous Subroutine</h3>
Message =
<!--#perl arg="Hello" arg="SSI" arg="World"
sub="sub {
my($r, @args) = @_;
print qq(@args);
}"
-->
<h3>Here is a Predefined Subroutine</h3>
Remote host = <!--#perl sub="MySSI::remote_host" -->
<hr>
</body>
</html>
That's all there is to it. You can mix and match any of the standard mod_include
commands in your document along with any Perl code that you see fit. There's
also an Apache::Include module included with the mod_perl
distribution that allows you to invoke Apache::Registry scripts directly
from within server-side includes. See Appendix A,
Standard Noncore Modules, for details. While this approach is simple, it is not particularly powerful. If you wish
to produce complex server-side include documents with conditional sections and
content derived from databases, we recommend that you explore HTML::Embperl,
Apache::ePerl, HTML::Mason, and other template-based systems
that can be found on CPAN. Also see Appendix F, HTML::Embperl--Embedding
Perl Code in HTML, which contains an abbreviated version of the HTML::Embperl
manual page, courtesy of Gerald Richter. Subclassing the Apache Class Show Contents Go to Top Previous Page Next Page
It's appropriate that the last topic we discuss in this chapter is the technique for extending the Apache class itself with Perl's subclassing mechanism. Because the Perl API is object-oriented, you are free to subclass the Apache class should you wish to override its behavior in any way.
To be successful, the new subclass must add Apache (or another Apache subclass) to its @ISA array. In addition, the subclass's new() method must return a blessed hash reference which contains either an r or _r key. This key must point to a bona fide Apache object.
Example 7-14 subclasses Apache, overriding
the print() and rflush() methods. The Apache::MyRequest::print
method does not send data directly to the client. Instead, it pushes all data
into an array reference inside the Apache::MyRequest object. When the
rflush() method is called, the SUPER class methods,
print and rflush, are called to actually send the data to
the client.
Example 7-14. Apache::MyRequest Is
a Subclass of Apache
package Apache::MyRequest;
use strict;
use Apache ();
use vars qw(@ISA);
@ISA = qw(Apache);
sub new {
my($class, $r) = @_;
$r ||= Apache->request;
return bless {
'_r' => $r,
'data' => [],
}, $class;
}
sub print {
my $self = shift;
push @{$self->{data}}, @_;
}
sub rflush {
my $self = shift;
$self->SUPER::print("MyDATA:\n", join "\n", @{$self->{data}}); $self->SUPER::rflush;
@{$self->{data}} = ();
}
1;
__END__
Here is an example of an Apache::Registry script that uses Apache::MyRequest. The send_http_header() method is inherited from the Apache class, while the print() and rflush() methods invoke those in the Apache::MyRequest class:
use Apache::MyRequest ();
sub handler {
my $r = Apache::MyRequest->new(shift);
$r->send_http_header('text/plain');
$r->print(qw(one two three));
$r->rflush;
...
}
The next chapter covers another important topic in the Apache Perl API: how
to control and customize the Apache configuration process so that modules can
implement first-class configuration directives of their own. Show Contents Go to Top Previous Page Next Page Copyright © 1999 by O'Reilly & Associates, Inc. |